Before anything, it must be noted that Native Ads are only available to certain providers Unless you’re one of them, you probably can’t make use of this (it pains me as well).
Native Ads are different. They’re versatile. You decide how their content is displayed. That makes them great. You can ensure they’re not disruptive to your users.
Where banner ads are small banners and interstitial ads are full screen ads, native ads work more like layouts that contain different views. You often see them in between content, blending well in.
As for the content, you decide how much content they show. They can show just text, or include a big fat image in there. It’s up to you and your desire for that sweet revenue. To do this, you build the ad with an AdLoader .
Implementing Native Ads is not so straightforward though. There is quite a bit to it, and here I’ll try to sum it up as simply as I can. This will build on knowledge from How to Monetise your Android App with Firebase Admob so if you don’t know how to initialise Admob with your app, you might want to read that first.
Adding the AdView in your layout
Native ads have two special AdView classes that correspond to the formats below: NativeAppInstallAdView and NativeContentAdView which act as ViewGroups. You should define another layout (say, linear/relative layout) as a child of the AdView that contains views for the assets your ads will use.
Such a layout could be like this. You should also define proper IDs to the asset views as you’ll be applying the data to the views yourself later on as well.
Ad Attribution 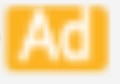
This is that small badge you see on the corner of ads that says “Ad” or “Advertisement”. It’s compulsory that you place this, and for Native Ads, you need to add these in manually by creating the view yourself. It doesn’t necessarily have to be a badge as long as it indicates that the view is an ad.
The view must be at least 15px width/height and must adhere to the guidelines stated here.
Native Test Ad
ca-app-pub-3940256099942544/2247696110
While developing, remember to use a test ad ID instead of a real one. Failure to do so could lead to the suspension of your account.
Two Formats for Native Ads
There are two different methods you can use to request two different ad formats. (You can choose to request both as well)
forAppInstallAd() – Makes the AdLoader request app install ads
forContentAd() – Makes the AdLoader request content ads.
For both of these methods, you pass on a listener for when the ad is loaded. These listener methods will trigger after you call loadAd (explained further below) which you’ll be doing after you build your AdLoader.
AdLoader adLoader = new AdLoader.Builder(context, "ca-app-pub-3940256099942544/2247696110") .forAppInstallAd(new OnAppInstallAdLoadedListener() { @Override public void onAppInstallAdLoaded(NativeAppInstallAd appInstallAd) { // Show the app install ad. } }) .forContentAd(new OnContentAdLoadedListener() { @Override public void onContentAdLoaded(NativeContentAd contentAd) { // Show the content ad. } }) .withAdListener(new AdListener() { @Override public void onAdFailedToLoad(int errorCode) { // Handle the failure by logging, altering the UI, and so on. } }) .withNativeAdOptions(new NativeAdOptions.Builder() // Methods in the NativeAdOptions.Builder class can be // used here to specify individual options settings. .build()) .build();
Setting the Options
You might notice in the code above the method withNativeAdOptions which allows you to set specific options while making the ad request. You pass a new NativeAdOptions.Builder which has these methods included:
setReturnUrlsForImageAssets() – While this is false (default value), the SDK fetches image assets automatically and populates both the Drawable and Uri for you. If set to true however, only the Uri field is populated by the SDK, allowing you to download the ad’s images at your discretion.
setImageOrientation() – The orientation for the ad’s image which can be set to ORIENTATION_PORTRAIT , ORIENTATION_LANDSCAPE , or ORIENTATION_ANY (default value).
This doesn’t eradicate ads with images that don’t match the set orientation, but instead, it priotises that those do. Not all ads have images in both orientation, so make sure your app can handle images at both orientations.
setRequestMultipleImages() – Some ads have multiple images. Setting it to true will allow them to display all those available images
setAdChoicesPlacement() – The AdChoices overlay is a small blue icon that is compulsorily placed on one of the ad’s corners. It’s set to ADCHOICES_TOP_RIGHT by default.
Loading Ads
Once you’re done preparing your AdLoader , you can choose to load a single ad with this method.
adLoader.loadAd(new AdRequest.Builder().build());
Or you can load multiple ads with this method
adLoader.loadAds(new AdRequest.Builder().build(), 3);
loadAds takes in the additional parameter of how many ads you want to load which is capped at a maximum of 5. Even with less than that, it’s not guaranteed that you’ll have that many ads loaded for you.
As said earlier, loading an ad will trigger the listener methods defined above, which are really just if it loaded or if there was an error. If you’re loading multiple ads, these methods will be triggered for each ad loaded/failed to load.
This means that in your onAppInstallAdLoaded or onContentAdLoaded methods, you should include these statements.
if (adLoader.isLoading()) { // The AdLoader is still loading ads. // Expect more adLoaded or onAdFailedToLoad callbacks. } else { // The AdLoader has finished loading ads. }
Inflating the AdView
private void displayAppInstallAd(ViewGroup parent, NativeAppInstallAd ad) { // Inflate a layout and add it to the parent ViewGroup. LayoutInflater inflater = (LayoutInflater) parent.getContext() .getSystemService(Context.LAYOUT_INFLATER_SERVICE); NativeAppInstallAdView adView = (NativeAppInstallAdView) inflater .inflate(R.layout.my_ad_layout, parent); // Locate the view that will hold the headline, set its text, and call the // NativeAppInstallAdView's setHeadlineView method to register it. TextView headlineView = adView.findViewById<TextView%>(R.id.ad_headline); headlineView.setText(ad.getHeadline()); adView.setHeadlineView(headlineView); // Repeat the above process for the other assets in the NativeAppInstallAd // using additional view objects (Buttons, ImageViews, etc). ... // Call the NativeAppInstallAdView's setNativeAd method to register the // NativeAdObject. adView.setNativeAd(ad); // Place the AdView into the parent. parent.addView(adView); }
When your AdLoader has finished loading the ad/ads, you can now apply the data from the ad to your asset views. You can create a method where you pass in the ad object from the listener method you used earlier.
You acquire the data from the ad object and apply it to your views as you would normally. Once all that is done, register the NativeAdObject then add the view and voila, you’re done! You’re ad should be displaying, but there’s one final thing you need to set up.
Destroying the Ad
nativeAd.destroy();
When you’re done showing the ad, you need to destroy it for proper garbage collection.
Conclusion
I told you it wasn’t going to be so straightforward, but I hope I put it clearly enough for you to understand. This article is really just this one from Google but simplified and summarised. This article didn’t mention anything about Native Video, so if you want to learn about that, check the Google’s article out!